0x0019 - Django - Setting Alternate DB Engines
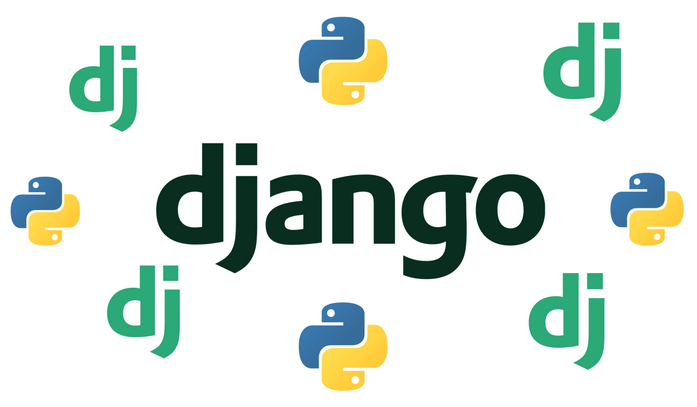
Inside settings.py
import os
BASE_DIR = os.path.dirname(os.path.dirname(os.path.abspath(__file__)))
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': os.path.join(BASE_DIR, 'db.sqlite3'),
}
}
Possible values are:
Engine type | Setting | pip install |
---|---|---|
MySQL | django.db.backends.mysql | pip install mysql-python |
Oracle | django.db.backends.oracle | pip install cx_Oracle |
PostgreSQL | django.db.backends.postgresql_psycopg2 | pip install ostcopg2 |
SQLite | django.db.backends.sqlite3 | NA - already installed |
A connection example is done inside the settings.py
DATABASES = {
'default': {
'NAME': 'my_database',
'ENGINE': 'sqlserver_ado',
'HOST': 'dbserver\\ss2012',
'USER': '',
'PASSWORD': '',
}
}
Another settings example:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'my_database',
'USER': 'root',
'PASSWORD': 'your_password',
'HOST': '127.0.0.1',
'PORT': '3306',
'OPTIONS': {
'init_command': "SET sql_mode='STRICT_TRANS_TABLES'"
}
}
}
Possible options:
Setting | Example | BreakDown |
---|---|---|
ATOMIC_REQUESTS | False | Forces a transaction for each view request |
AUTOCOMMIT | True | Faster as the SQL undo is off |
ENGINE | django.db.backends.mysql | Specify the engine that you are using |
HOST | 192.168.2.1 | Or the www.domain where your server sits |
NAME | Database_name | Specify the database name to use |
OPTIONS | {} | A list of parameters |
PASSWORD | 'your_password' | |
PORT | 3306 | Typical port to use |
USER | root | SQL access user |