0x001B - Django - Template Basics
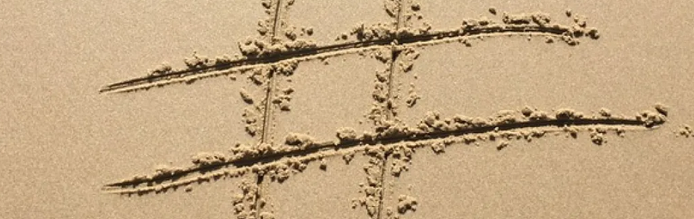
Next we are going to look at template basics - which is possibly the most powerful feature of Django (guide)
- To render a template one needs a view and a URL mapped to that view. geeks/view.py
#import HTTP response from django
from django.shortcuts import render
#create function
def geeks_view(request):
context = {
"data": "This is the data field",
"list": [1,2,3,4]}
return render(request, "geeks.html", context)
Now we will map this to a view:
from django.urls import path
from .views import geeks_view
urlpatterns = [
path('', geeks_view)
]
Now the template which was referenced above geeks.html is connected the to the created context.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Homepage</title>
</head>
<body>
<h1> Test page </h1>
<p> Data is {{ data }}</p>
<h4>List is </h4>
<ul>
{% for i in list %}
<li>{{ i }}</li>
{% endfor %}
</body>
</html>
- Some constructs are recognized and interpretated by the template engine.
Variables:
- {{ first_name }}
- {{ last_name }}
Tags:
- if: {% if my_list %} if the object exists is not empty. Closed with a {% endif %} tag.
Expansive testing is also supported here, for instance we may:
{% if somevar == 9 %}
This string appears if the above is True!
{% endif %}
- for iteration: {% for b in my_list %} each iteration is then referenced with a {{ b }}. Finally it is closed with a {% endfor %}
- now: {% now "D M Y H T " %} expressing the current datetime in the specified format.. Variable construction is also allowed as in: {% now "Y" as current_year %} which is then referenced later as in {{ current_year }}
- include: {% include "inside_html.html" %} which loads the next template inside the current template thus it too can be rendered.
Filters:
- autoescape: {% autoescape on %} {{ body }} {% endautoescape %} Turn on/off HTML escaping.
How this works is if you are 'html-escaping' you will 'pass-through' your html tags so they become visible to the web page. If you had <h4>my block</h4> it would normally show up as my block but if html escaping is turned on you will see out the end <h4> my block </h4>
- comment: {% comment "Note" %} <p> This code is shut off </p> {% endcomment %}
- filter: {% filter force_escape | lower %} This test will be HTML-escaped, and will appear in all lowercase. {% endfilter %}
- firstof: {% firstof var1 var2 var 3 %} Will output the first non-empty existing variable from the iteration of var1, var2, var3.