0x001F - Java CSV Parser Example
Java CSV Parser Example
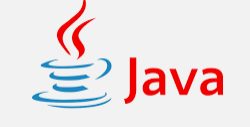
In this case we have built a CSV reader to read 1 minute data from a file:
- Starting offset is set by int startat,
- CSV files may return extra/empy row sets. These are filtered for in the while (line != null)
- String G[] creates a string array from the line.split(",")
- Java does not have structs similar to C++, instead for each object we create a sub-class at the bottom with a initializer public Quote(float open, float high, float low, float close, float volw, float vol, long ntrades, LocalDateTime dtime)
- Quote data for the timestamp is in Epoch milliseconds or number of milliseconds after Epoch which for computers is 1970-01-01 00:00. The code LocalDateTime datetime = Instant.ofEpochMilli(toffset).atZone(ZoneId.systemDefault()).toLocalDateTime(); does the conversion.
public List<Quote> csv_process(String tfile, int startat) throws IOException
{
List<Quote> quotes = new ArrayList<Quote>();
Path pathToFile = Paths.get(tfile);
try (BufferedReader breader = Files.newBufferedReader(pathToFile, StandardCharsets.UTF_8))
{
String line = "";
int c = 0;
while (c < startat)
{
line = breader.readLine();
c++;
}
while (line != null)
{
line = breader.readLine();
if (line != null) {
String G[] = line.split(",");
if (G.length >= 8) {
try {
c++;
float open = parseFloat(G[0]);
float high = parseFloat(G[1]);
float low = parseFloat(G[2]);
float close = parseFloat(G[3]);
float volw = parseFloat(G[4]);
long vol = Integer.parseInt(G[5]);
long ntrades = Integer.parseInt(G[6]);
long toffset = Long.parseLong(G[7]);
LocalDateTime datetime = Instant.ofEpochMilli(toffset).atZone(ZoneId.systemDefault()).toLocalDateTime();
Quote newquote = new Quote(open, high, low, close, volw, vol, ntrades, datetime);
quotes.add(newquote);
} catch (Exception e) {
}
}
}
}
} catch (IOException ioe) {
ioe.printStackTrace();
}
return quotes;
}
}
class Quote {
public float open;
public float high;
public float low;
public float close;
public float volw;
public float vol;
public long ntrades;
public LocalDateTime dtime;
public Quote(float open, float high, float low, float close, float volw, float vol, long ntrades, LocalDateTime dtime)
{
this.open = open;
this.high = high;
this.low = low;
this.close = close;
this.volw = volw;
this.vol = vol;
this.ntrades = ntrades;
this.dtime = dtime;
}
}
Once this is done we return quotes which is of type List<Quote> which is added in lines:
Quote newquote = new Quote(open, high, low, close, volw, vol, ntrades, datetime);
quotes.add(newquote);